 Thursday, 15 September 2011
Word is starting to get out about C++ AMP, which appeared out of nowhere at a conference remarkably few Microsoft developers were paying attention to, because it was a hardware conference. There was information available in June, enough to get some of us excited: I got into this right away and have been playing with code and doing a little writing. This is the kind of technology that changes things more than you might think. By leveraging the GPU, your code might run 10x faster, 50x faster, or even 100x faster. And for you to be able to do that from C++, using familiar C++ constructs, and a debugger and profiler in Visual Studio? That means everyone can do it. Well, not quite everyone. You do have to learn how to parallelize your algorithms. The syntax of using the GPU (or some other heterogeneous computing resource) is not hard at all. The computer science of knowing your work is data parallel can be hard. But let me show you "not hard". Consider this code to add a pair of one-dimensional array: void AddArrays(int n, int* pA, int* pB, int* pC) { for (int i=0; i<n; i++) { pC[i] = pA[i] + pB[i]; } }
Compare that to this: #include <amp.h> using namespace concurrency; void AddArrays(int n, int * pA, int * pB, int * pC) { array_view<int,1> a(n, pA); array_view<int,1> b(n, pB); array_view<int,1> c(n, pC); parallel_for_each( c.grid, [=](index<1> idx) restrict(direct3d) { c[idx] = a[idx] + b[idx]; } ); }
It's all C++ and it's all pretty readable. And this code runs on the GPU and can be WAY faster (and use less power, meaning your data centre is cheaper or your battery lasts longer) just like that. Recently Daniel Moth has published ten blog posts drilling into some details. They will help if you've decided to start using AMP and want to know how. But before you do that, you might like to read a little background on why heterogeneous computing matters, what other options you might have for doing it, and why C++ AMP is what you want to use. I've done a small whitepaper on just that and would love you to read it and let me know what you think. Kate
 Tuesday, 13 September 2011
I've been writing a course for Pluralsight that covers the fundamentals of C++ - types, expressions, basic syntax stuff, templates, pointers, polymorphism - and it has gone live! During BUILD access to it is free. It uses Visual C++ Express, so you don't need to buy any tools to follow along. Lots of buzz at BUILD about C++, so if you want to see if it's a language you could use, here's a great way to find out. I have more material coming on this, and some Windows 8 - specific material. This is just background. If you think you need to get up to speed on C++, here's how to do it. Let me know what you think. Kate
 Monday, 29 August 2011
For a long time now, whenever anyone asks me about support for particular C++0x (oh sorry C++11  ) features in a given compiler, I've sent them to the list Scott Meyers maintains. The format is kind of strange because it's exported from a spreadsheet, but the information is invaluable. If you'd like to see more compilers covered, try the wiki at apache. Scott does gcc and Microsoft Visual C++; the wiki adds compilers from Intel, IBM, Oracle, and so on. You might be surprised to see the variations in coverage. Expect new versions to continue to add support as soon as possible. Kate
 Saturday, 27 August 2011
I was poking around on CodeProject looking at some developer interviews (they've started these up again with some interesting subjects, so check the recent links) when I spotted An interview with Microsoft's new
Visual C++ .NET community liaison - and in March 2002, nearly a decade ago, that was Herb Sutter. Isn't the Internet a handy attic? Check out some of these quotes: I hope to make a noticeable mark in the product.
C++ continues to be relevant, dominant, and in widespread and still-growing
use. The C++ standard and standardization process also continues to be relevant [...] all the vendors, including
Microsoft, are there together actively working on the next-generation C++0x
standard whose work is now getting underway.
[...] the best numbers I keep seeing put the global
developer community at something like 9.5 million people, and those using C++ at
about 3 million of that. That's well ahead of Java in nearly all studies I've
seen, by the way, usually by a factor of 1.5-to-1 or 2-to-1. [...] The reports of C++'s demise have been, well,
"exaggerated."
C++ developers need power and know how to use it. I've always said you should
use the best language for the job, and I've used dozens of languages
professionally. Depending on how you count languages, I've probably used a dozen
professionally in the past year. People who want to write efficient, tight, fast
code often tend to choose C++ because it lets you get the job done with powerful
code but without sacrificing efficiency.
People who want mature, stable compilers and tools often tend to use C++
because it's been around a while and the tools and libraries are plentiful and
solid. Commercial client-side application development with more than a few
screens, most kinds of server-based software, and most kinds of libraries are
all done more often with C++ than with other languages, according to the best
numbers I've seen and according to my own experience as a developer and as a
consultant who shows up at other developers' shops. Nine and a half years later, I see nothing but good stuff there. I hope all my interviews stand up as well (though I already know they wouldn't) and I'm impressed at the ability to set a goal and meet it. What will the next decade bring? Kate
 Thursday, 25 August 2011
The cone of silence that descended this summer is starting to fray a little. The Visual C++ Team Blog has an entry talking about Productivity features in the IDE. They're willing to talk about: - Semantic colorization - this is actually as much font face as it is colour, but anyway function parameters look different from locals, constants you #define'd look different from everything else, and so on.
- Reference Highlighting - you've seen this in other languages I'm sure - when the cursor is in a variable name, other places in the code with that same variable name are highlighted for you. It can be very helpful.
- Replacement Solution Explorer - I'm a huge Pro Power Tools fan, and if you've used Solution Navigator, you won't be surprised when you meet the new Solution Explorer. Combination Solution Explorer and Class View is the best way to explain it to a C++ developer.
- Aggressive IntelliSense - something else you may be familiar with from other languages. I like it.
- Snippets - oh, yeah!
Apparently there's still plenty more to come! Looking forward to it.
 Tuesday, 23 August 2011
C++ is a great language for writing applications that will run on a number of platforms. There are compilers for many different platforms, and some powerful libraries you can use. Still, in the end your code needs to talk to the operating system, and that means that most cross platform applications have at least a few little corners where platform-specific code lives. The challenge is how to ensure that your Windows code runs on Windows, your Linux code runs on Linux, etc, without maintaining a number of different branches or hand-merging and splitting every time you deploy. Being C++, an unspoken requirement in solving this problem is "be as fast as possible". Putting everything through a library and paying for extra indirection, looking up something that cannot change once the program has started executing, is not as fast as possible. You also want developer convenience and productivity. If you support five platforms, and something is the same on four and different on one, copying that code around for the four that are the same is not a productive way to behave. You would like a default behaviour, and then special code for special cases. Michael Tedder has an intriguing approach using templates. As he says: Instead of declaring a base interface class with virtual
functions then deriving each platform with a different implementation, we
declare a class with one template parameter — a platform ID — then specialize it
to provide a different implementation for each platform. The template class is
then typedef ‘d to expose the specialization for the platform ID
being compiled to the application, allowing the implementation to be used
without any virtual functions and also allow for inlining of functions as
well.
He has some pretty convincing armwaving about using this not for just Windows/Linux/Android but for any hardware differences even on the same operating system - like what kind of graphics architecture you have or anything else that can't change at runtime. It's a good example of how the power of templates makes things possible that would always incur a runtime cost in any other language, or a significant burden on a developer to move code around building custom versions of an application. Worth a read! Kate
 Sunday, 21 August 2011
Here's an interesting project I came across: 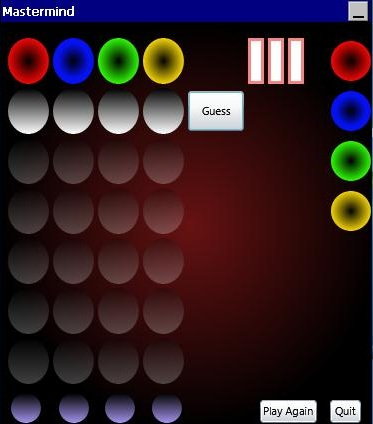 The game is Mastermind, which many people already know. What caught my attention is that it's done in C++ and XAML. To quote Tyler Whitney's blog post, The Mastermind code sample demonstrates how to create a simple Silverlight
storyboard in Expression Blend 3, and then provide the code for the state
transitions in Microsoft Visual Studio 2008. You can also see how to create
brushes programmatically and how to add a title bar to a Silverlight-based app.
The code is on the Microsoft Code Gallery (only Microsoft Employees can publish there) and Tyler has been updating the article over the summer. Nice way to see how to combine two technologies some people would never thought have combining. Kate
 Friday, 19 August 2011
I have talked about plenty of C++0x (soon to be known as C++11 or just plain C++) features over the last little while. Here's a nice summary by Danny Kalev of the top features and why they matter. He covers lambdas, auto, the new ways to initialize instances (which hasn't been covered much elsewhere and contribute a lot to readability), suppressing default versions of functions (like constructors for example) or specifically requesting them (how cool is that?), nullptr (which I love because it eliminates a late night drinking argument about "what if someone #defined NULL to 3, would your code still work?", and rvalue references -- and those are just the language changes! His library coverage is super terse, but there are links in it if something (*cough* shared pointer *cough*) catches your attention. There's no reason for a C++ developer to ignore C++11. This is big stuff, and reading these "what's new" lists from a variety of different people is essential for getting perspective on the changes. So read Danny's list! Kate
© Copyright 2024 Kate Gregory
Theme design by Bryan Bell
newtelligence dasBlog 2.3.9074.18820  | Page rendered at Friday, 26 July 2024 20:24:54 (Eastern Daylight Time, UTC-04:00)
|
On this page....
| Sun | Mon | Tue | Wed | Thu | Fri | Sat |
---|
28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
Pluralsight Free Trial
Search
Navigation
Categories
Blogroll
Sign In
|