 Thursday, 28 May 2020
Lately a lot of people have been asking me for help as they write C++ code. I’m usually happy and able to help. There are times, though, when I either cannot help, or choose not to help. I thought it might be helpful to explain some of these a little. It’s quite likely that other members of the community have a similar set of guidelines in their heads for when they do and don’t help people with code. Warning: this is long. I think it's worth reading it all. You want a TL;DR? It’s this: if you want free mentoring and consulting from successful people, you can have it! All you need to do is ask. But if you expect your helpers to do most of the work in helping you, and to take instruction and direction from you, you’re going to get a lot less help than if you know how to be helped effectively. The first choice is where and how you ask. People often email me, message me on LinkedIn, DM me on Twitter, DM me on the #include <C++> Discord, and so on hoping to get personalized, instant, one-on-one help from me. That’s not a good use of my time. I prefer to help in places where others will see the question and the answer. That helps more people. It also enables more people to help – so it produces better advice as well as helping those people learn and grow. Often, I learn from the times when other people chime in. So I encourage you to post on StackOverflow (if you have the sort of just-one-question, just-one-right-answer problem that fits there), or on the #include <C++> Discord, or some other public place where a number of people can see the question and the answer over time. The rest of the issues have to do with how much work the person expects me to do, or how much they save me. Think about how you ask the very first thing you ask. Compare “here is a zip of all the files in my project can you tell me what’s wrong with it?” to “here is a link to an online compiler (Godbolt, wandbox, etc) showing a compiler error on line 43 that I don’t understand. Can you tell me how to fix that error?” Expecting someone to install things, trawl through multiple files, guess your question, and then solve your problem and explain it all to you is really too much. At least tell people what your problem is! Often people ask for help saying something like “it’s not working” or “what’s wrong with this?” and I don’t even know if it’s a compiler error or a runtime error or running fine but calculating the wrong answer. So before you post on the discord, for example, try to have a single crisp question, not just “help, it’s not working.” - Show us your code, and your errors. And not as screenshots! You can copy the code into an online compiler like Godbolt or Wandbox, or if it’s less than 20 lines or so, paste it into the chat window – but please learn how to format it as code when you do that. Copy and paste the errors as text. That makes them much easier to read, and lets us copy and paste parts of them while explaining things to you. If you can’t show your real code because of how big it is or because it’s work-related, create a tiny example that shows the same problem and show us that. Stack Overflow has some tips on how to do that. Whatever you do, do not free-type some code into the chat window that you think is the same as your problem, and then whenever people point out missing semi colons or undeclared variables, reply “oh yeah, that’s not really my code it’s just something I typed to give you the general idea.” Compilers (and runtimes) are picky and asking for help with something that’s vaguely like your real code (but not really) is pointless.
Now let’s assume you manage to get a conversation going with someone who is trying to help you. They solved that compiler error, for example, but now you have another one. As part of this process, I often make suggestions to people that they reject. I think they believe the suggestions are to make things easier for themselves in the long run, because they say things like “I can do that once it’s working.” I then have to spend a lot of effort explaining that I want them to do these things so that I can help them get it working. These suggestions include: - Write good variable (and function) names. If your variables are all called i, n, c, r, s, and so on – I don’t know what they represent. If I ask you to change those to words like next, rate, total, and so on, or to words you think of yourself, that’s because I can’t understand your code (I don’t know the problem you’re trying to solve) without some help. Good names aren’t a someday thing that you paint over working code once it’s all good. They are how you make code other people can read. And you’re asking me to read this code. Make it readable.
- Use a debugger. When I ask “have you looked in the debugger to see the value of a before the loop?” an answer of “I don’t know how to use the debugger and I don’t have time to learn that today” is a great way to end our conversation. Real programmers use the debugger. We don’t have some magical compiler-simulator in our heads that can read code and tell you if it compiles or not, and we don’t have a magical runtime-simulator either. Sure, maybe I can tell at a glance that a is 0 before the loop and that’s why it’s not working, but in that case I would tell you so. It’s more likely that I want you to quickly check and see if it’s 0 or not. When you refuse to debug, you’re making it so much harder to help you. You need to learn to use whatever debugger is available to you, and you will probably save enough time today to make up for the time it takes to learn it.
- Add some tests. You don’t have to go learn a whole unit testing framework. But if you’re writing a function to do whatever, work out by hand what it does for simple values, and write a test harness that passes it those simple values. Then you can debug the test harness and see what the function returns and confirm whether or not it works for simple values. Whether you’re reversing a string in place, calculating the Fibonacci sequence, calculating sums of things, whatever, you should be able to think up simple test cases and test your code with them. And eventually, you should be writing tests as you write your code. It’s a good habit you can build now.
- Break up big things. You don’t have to embrace full OO or write functional programs, but don’t give me 1000 lines of code and ask me to load it into my head. Write some functions. Heck, throw in some comments and some blank lines. Show the structure of your code so it’s not a wall of text.
Some other good behaviours that will take you a long way: - Try the substitutions people tell you to try. A lot of times, people who are having a hard time don’t want to learn a new thing. I run into that situation all the time myself. I’m already frustrated and I’ve spent longer than I meant to and I can’t understand any of it, I don’t want you to tell me to go learn yet another thing right now. I have gained some wisdom over the years though, and it includes this: sometimes jettisoning all that half-understood not-really-working mess and doing something simpler is the best way forward. If someone tells you that vector would be better here, and offers you a few lines of code to use, just digging in your heels and refusing to try it isn’t going to lead you into learning. If you’ve got a problem because you’re trying to manage memory yourself by hand but you forgot about copying and so on, then using a smart pointer, or dropping the pointers altogether and using an object on the stack, is going to make a whole pile of work just fall away. The person advising you to try this knows how much effort it will save. You don’t, that’s why you came for help. It’s really frustrating to see a beginner insist on doing something the hard way (for no benefit), do it wrong, and refuse to accept any help other than “here is the precise and exact code to do that thing the hard way.” I don’t want to do things the hard way any more: why would I type out all the code for you?
- Try things that don’t matter to you, if the person who is helping you tells you that your code is harder to read the way you have it. Things like initializing member variables in a constructor with the : syntax, not between the braces, or adding some using statements – these may not matter to you, but making things too hard for a busy helper may mean that helper is too busy to help today. Or ever. I don’t want to teach you bad habits, I don’t want to teach you to “pretty things up” only once it’s working, and I don’t want to exhaust myself reading difficult code to spare you the trouble of doing the right thing. Also, when a person asks for advice but never takes any of it because they’re sure it’s not actually relevant to their problem, eventually the advice-giver will stop giving it. It’s pointless.
- Write your own code. If I tell you “the problem is that you’re not initializing x” don’t ask me to edit your code for you or paste in the new version of the function or whatever. You need to understand what you’re doing and that comes from writing the code yourself. If you don’t understand how to fix a problem that someone has told you about, ask them “how do I fix that?” If you can’t understand their answer, say “I don’t know what [whatever] is, can you explain it or show me?” Don’t just ask “what would that line of code look like?” That feels like you’re asking me to even do the typing for you.
- Work with whoever is talking to you. Maybe when you first ask, one person has a couple of thoughts, and those are good, but while you’re changing your code to see if that works, someone else chimes in. That’s great. It’s a group chat. Don’t tell them that you’re working with the first person or anything like that to reject their help. Consider all the suggestions you get. If you’re talking to someone and then they stop, that’s cool too. Many people pop into chat for 5 or 10 minutes waiting for a conference call to start, or while they’re eating lunch, and don’t stay long. People get called away from their keyboards. Don’t start pinging the person trying to bring them back or ask if they have any more thoughts or saying you’re still stuck. You can tell the room or channel as a whole that you’re still stuck. Maybe someone else will have some ideas. Your problem may end up solved over an hour or so with three different people. That’s a win!
I know, that’s a lot of advice. Thing is, you can get a lot of help from strangers on the internet, if you ask the right way. If you ask the wrong way, most people will just shrug and say “looks like you have a problem” and move along. They won’t even tell you why they’re not helping you! To get the marvelous free help, and to truly join the community, you have to put in a little effort. Trust me, it’s worth it! Kate
 Wednesday, 06 May 2020
Since late February, when I returned from a personal trip to Singapore, my travel and conference world has been shrinking in around me. Conference after conference has been cancelled (or postponed to next year which is the same thing), moved online, or put off to perhaps later this year. Of course, the rest of my world has also been shrinking: for the last 8 weeks I've left the house only a handful of times, and seen almost no-one. I'm sure it's the same for you. So it was quite a surprise to remember that my last conference wasn't actually that long ago: the video of it has just gone live. This is a shortened version of Emotional Code for students, who don't all know C++ and don't all have a lot of experience with other people's code. I hope you like it. I've also updated my playlist, which has all the talk recordings I know about. If you're looking for conference substitutes around now, perhaps there's a talk of mine you haven't yet seen? Take a look at it now. Looking forward to in-person conferences and live audio feedback once again,
Kate
 Sunday, 19 January 2020
This week I travelled to Montreal to deliver a keynote at CUSEC 2020, the Canadian University Software Engineering Conference. Everything was nicely arranged and I happily took the train from Oshawa to Montreal, then a short all-inside walk to the hotel where I checked in, told the organizers I was there, and settled in for an early night. I got a light dinner from room service but oddly could only eat half of it. Ah well, I thought, they fed us really well on the train, I'm probably just full. No big deal. I went to sleep. About 10:30 I woke up and realized I needed to throw up. So I did. And did. And did. All night. For an extended part of the night it was every 45 minutes. It was bad. And then it got worse. Now I am not telling you this to gross you out or to overshare, but to get you, as a possible speaker or conference organizer, to consider this possibility if you have not done so before (I had not.) I felt perfectly normal when I left home, and even when I first arrived in town. Whatever food poisoning or virus got me, it hit fast and hard. When the sun finally dragged itself up over the Montreal horizon and into my eyes, I was exhausted, having not slept all night, and pretty sure I was not done throwing up (which it turns out I was not.) I got on Slack with my organizers and told them I could handle being tired but actually vomiting while on stage was a bridge too far for me. Could we switch with someone scheduled for Day 2? Of course we could. They did that lovely duck trick, where above the water it all looks smooth and simple and you have no idea what amount of paddling and ruddering is happening underwater. Someone else did an opening keynote; my keynote moved to 11 am Day 2. A much needed bottle of ginger ale appeared at my door. I spent the day in bed and slowly returned to normal. I slept that night and did the keynote the next day, and very much enjoyed the rest of the conference. I didn't shake hands with anyone in case I was contagious. When the AV people started touching my laptop I gave them hand sanitizer.
So, if this happened to you, would you be able to come up with a plan B? Do you travel with anti-nausea meds? (I do, for airsickness, and took some to help me sleep during the day since they sedate me. They had no hope of working during the worst of it, but they still had value.) Do you have a little bottle of hand sanitizer with you all the time? (I do, and always will.) Do you know how to reach your organizers with some urgency when you can't leave your room? Organizers, I hope you would all react as smoothly and quickly as my CUSEC hosts did. Ellen and Afreen were ultra professional, as was everyone else I dealt with. You don't want to think about it, I know. But -- you should, anyway. It doesn't take long to have a disaster recovery plan. Swapping two keynotes was the obvious choice, and it worked because the keynoters were staying for the whole conference not just popping in for their morning. A little prior preparation can predict proper performance, or something like that. Kate
 Wednesday, 08 January 2020
Every once in a while, I make a big change in how I plan and manage my speaking engagements. Early in the last decade, I decided to speak only at conferences I would happily pay to attend, and that improved my life dramatically. Instead of trying to justify a week away from home and the office in which I would try to keep up on emails from a hotel room or a hallway couch, while surrounded by people who didn't care about the stuff I cared about, I started looking forward to a week of learning and growing, of coming home knowing more than when I left, and of meeting my heroes and getting to see my friends. While this was an important change, it was only a change in my decision criteria, and not in my overall process of deciding where to speak. I would get an email, or see a tweet, or otherwise become aware that a conference was going to happen, and then I would decide, on a case-by-case basis, if I wanted to submit to that conference. Sometimes I would have to decline because I had already submitted to another one at roughly the same time, without realizing the overlap. In mid 2019, I changed that. I listed out all the C++ conferences I knew of, and roughly when they happened. Then my partner and I went through the list, noting when various family events are happening, when we want to go on vacation, and other "big rocks" that conferences have to fit around. We talked about how many conferences I wanted to speak at, and whittled down the list to that many. Now, as each conference opens a Call for Papers, if it's on my list, I submit, and if it's not, I don't. Of course, my talks aren't always accepted. I set myself a goal to speak at two non-C++ conferences in 2020. I was invited to one, but after I agreed they changed their dates and that conflicted with something else I had accepted. I submitted to another and they declined my talk. But one has accepted, and I have accepted another invitation, so I will be speaking at two non-C++ conferences for sure. Expect to see me at: - January, Montreal: CUSEC 2020 (Canadian University Software Engineering Conference) - keynote (and a Meetup while I'm in town, come ask about technical speaking)
- March, Bristol, UK: ACCU - Naming Is Hard, Let's Do Better
- May, London, UK: SDD - Naming and Emotional Code
I have submitted to some for June and onwards, but
haven't heard, so I'm not mentioning them, nor the ones I've decided not to submit to. That's not
fair to anyone. I might do as many as 7 conferences by the time the year is over, and that's a lot. Plus user group talks whenever I can.
So is there any point inviting me to speak at your conference? Well, sure. It might match up with something else (at least one conference I added to my list because I could combine it with another trip that was already planned) or be so compelling that I will find a way to fit it in. Or it might end up on my list for next year -- I like this advance planning so I'm going to keep doing it. As always, remember that I do have requirements for any speaking engagement, so if you invite me, please let me know you've read that and meet them.
If you're at any conference I am speaking at, please do find me and say hi! It's one of the most important parts of any conference for me.
Kate
 Thursday, 28 November 2019
I'm thrilled to announce my latest Pluralsight course: 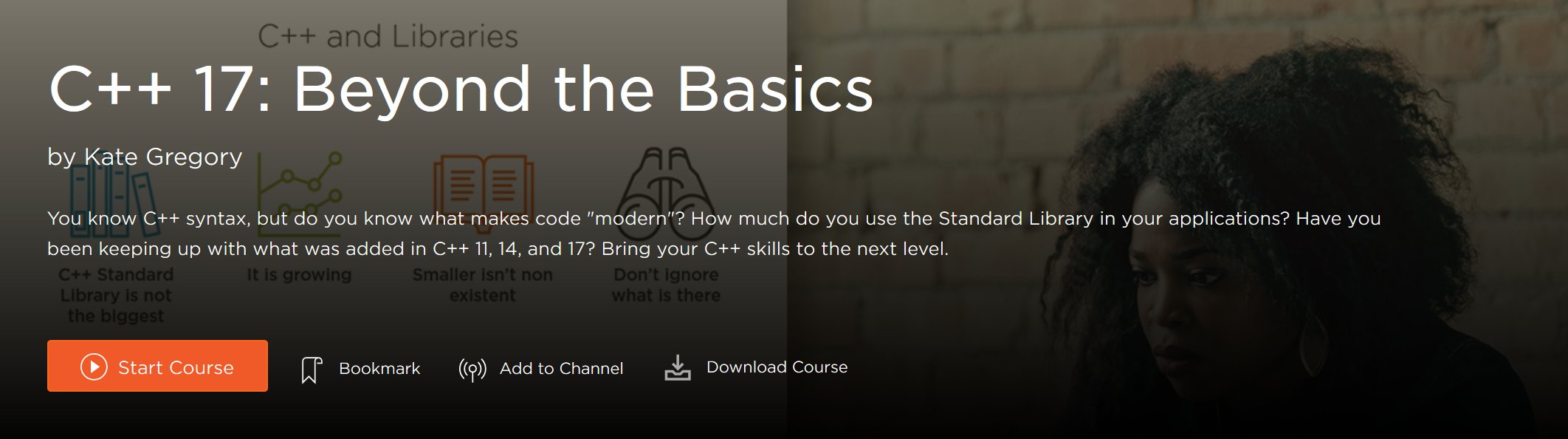
Here are the modules: - Course Overview
- Modern C++
- Standard Library Containers
- Lambdas
- Standard Library Algorithms
- Move Semantics
- Exceptions
Hope you enjoy it!
Kate
 Thursday, 25 July 2019
CppCon is approaching again and my calendar is FULL. The schedule may change and there are things still to be added, but the current plan is: - On Sunday, I am doing a preconference workshop (you can still get a spot) with John Lakos and Andrei Alexandrescu
- After the precon I hope to relax at the TShirt dinner.
Pack a Tshirt that says C++ (or bring your badge, or a piece of paper
on which you wrote C++ yourself) and choose a restaurant from the list that should be published by the time we all get to Colorado. When you arrive, ask where the other C++ Tshirt people
are, and make some new friends! I'll be tweeting my plans just before I
head out.
- Then it's back to the shiny new venue for the Registration Reception.
Even if you're on East Coast time like me, make an appearance, see some
friends or some of your heroes, there will probably be treats, and you'll
be all set for the morning. Knowing the venue a bit is going to make
you sleep better, and having your badge already will let you sleep
longer.
- Monday I will be attending talks and spending time at the exhibitor table for #include<C++>, an organization working to make the C++ community more welcoming and inclusive. Come by and get a sticker! Buy a shirt! And you know, attending talks and hanging at the #include table is what I'll be doing all 5 days.
- If I manage to stay awake, I'll go to The Committee Fireside Chat after dinner. If there is something you always wanted to ask the people who create the C++ standard, here's where that happens.
- Tuesday and Wednesday it's talks, talks, talks! I predict I will go back to my room for a nap at least once. It's a strategic choice that lets you actually experience the post-nap talks instead of drowsing through them and needing to watch the recording in the end.
- Wednesday night is the #include<C++> dinner and panel! You can register for this on Eventbrite as part of registering for the conference. I'll moderate a discussion about some of what we've achieved in just two years, and what some of us would like to see next.
- Afterwards it's Lightning Talks. Everyone loves the Lightning Talks, they're always fantastic. I will try my best to stay up for them.
- If I can, I'll come super early on Thursday and Friday for recordings of CppChat. And stay all day for talks, of course.
- Thursday night is the Speaker's Dinner. And there's a planning meeting after that. But I might need an early night, because...
- Friday morning I have my one breakout session: Naming is Hard: Let's Do Better. And like last year, a lot of really good content is on Friday. Don't even think of leaving early. Fly home Saturday morning, you won't regret it.
See why I call CppCon
an intense conference? 12 or 13 hours a day, every day. And no time for sightseeing! But oh my
goodness the things I will learn, the people I will meet, and the fun I
will have. See you there! Kate
 Sunday, 21 July 2019
July 21st, 1969 I (with my parents) moved to Canada for the second time. The first time, I had been an infant, and the move had been temporary: my father was doing his PhD at Carleton University in Ottawa. When he completed it, we went back to England as the plan had always been. We had grown from a family of 3 to a family of 4 in the meantime. But when they got back to England, they missed Canada a lot. So, by 1969, they had found a way for the now 5 of us to return. Originally it was to Ottawa and a job in a government research lab for my father. But within less than a year he decided to take a chance on the very new University of Waterloo and he worked there (with consulting clients and inventions and other side projects) until he retired to Nova Scotia. I often warn friends who are considering emigrating that if you do, you are likely to raise children who think emigrating is ok: my Canadian-born brother lived in Japan, Europe, and the US for decades before settling in Vancouver. My UK-born sister has been in Ireland, England, and now Wales for a similar length of time. I have two other sisters and one of them has also changed continents a few times and now lives in England. Me, I've stayed put. I like it here and couldn't imagine living anywhere other than the Ontario countryside, though I sure do like to visit other places! I remember very little of the move and the change of countries. We had been told of the rabid animals (there is no rabies in England) and the importance therefore of never letting a squirrel or chipmunk near you. Also of the cold, which I didn't remember from my first time. While I can't remember any of the moon landing hoopla at all, I do remember one scene from the airport as we arrived. We approached two doors, one for Canadians and one for all others. Well not doors, more like archways in a wall. And some official insisted that my brother go through the Canadian archway. He would have been 6 or 7 and my parents resisted but this official was adamant and said it would be fine. Which it was, because there was no wall between the two areas so it was a separation of a minute or so in full sight. We went through the arches at the same time but when we got through, this official knelt down to my brother's height and said "welcome home, little man" to him. I have other memories, of being bullied at school for my accent, of clashes with teachers who literally refused to teach me things I didn't know because "we covered that last year", of amazing beauty and nature and discoveries of all kinds, but that moment is one of those that really sticks with me. This place is home for me too. Kate
 Sunday, 14 July 2019
On July 14th, 2017, Guy Davidson tweeted what he thought was a passing pun: 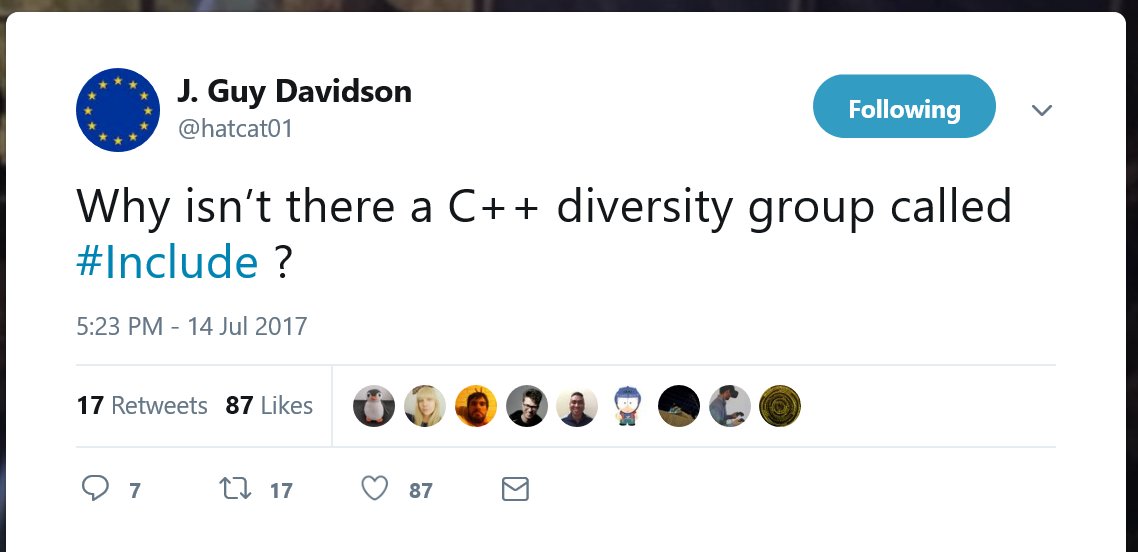
But when I saw the tweet, I thought, yeah, why isn’t there?
And in that moment, #include was born. We got together at CppCon and again at Meeting C++ where Guy did a lightning talk on inclusion. We were off and running. At that time we had a channel on the cpplang slack, but that didn’t work out well: a change of owners of the slack to someone who was less interested in preventing harassment and abuse, coupled with slack’s fundamental design tenet that people having trouble with bad behavior on a slack channel can always go to their mutual boss (which doesn’t work on public servers that bring strangers together) resulted in #include being pretty much driven off the slack and forming our own server elsewhere, on discord. The original channels on the discord were all about the work of running #include. How can we get conferences to have a code of conduct? How can we help employers to write job ads that will attract all kinds of applicants, not just people who closely resemble the ones they already have? But we couldn’t stop talking about C++ so we added a channel for that, and then another for something else technical, and another, … and things really started to grow. By April 2018 we were about a dozen organizers and very few people who weren’t organizers. But now we have over 2300 members and over 70 channels. People are getting help with C++ problems they face, recruiting helpers for projects, getting advice about speaking or attending conferences, and much more. Our original goals were pretty low key really: - To encourage under-represented people to speak, to apply for jobs, to stay in this industry
- To get conferences to have a code of conduct (we hadn’t even thought about enforcement)
- To get employers to value diversity somewhat, and to provide some resources to conferences and employers
We thought it would be nice to have some stickers and Tshirts made, and have a table at conferences where we would urge people to join our discord and try to make our industry more welcoming. Well, that worked! We’ve had tables at major C++ conferences the world over and you can be sure to find a smiling person to talk to, whether they’re officially “working the table” or not. 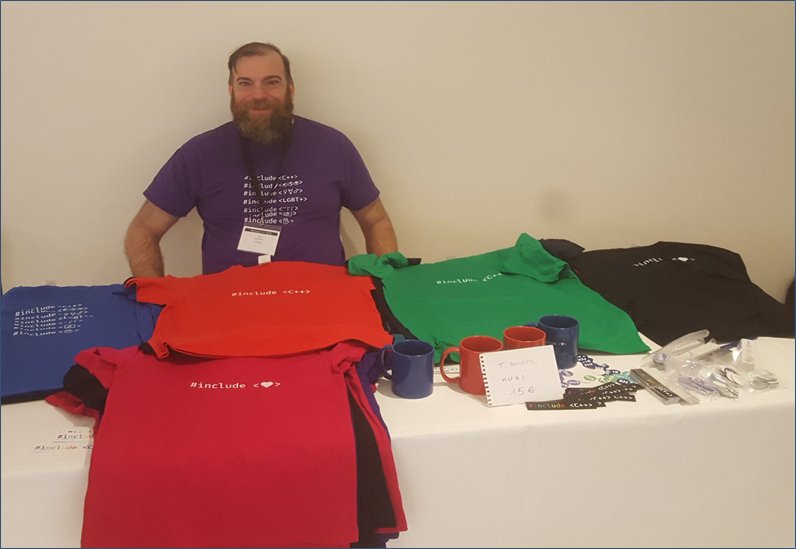
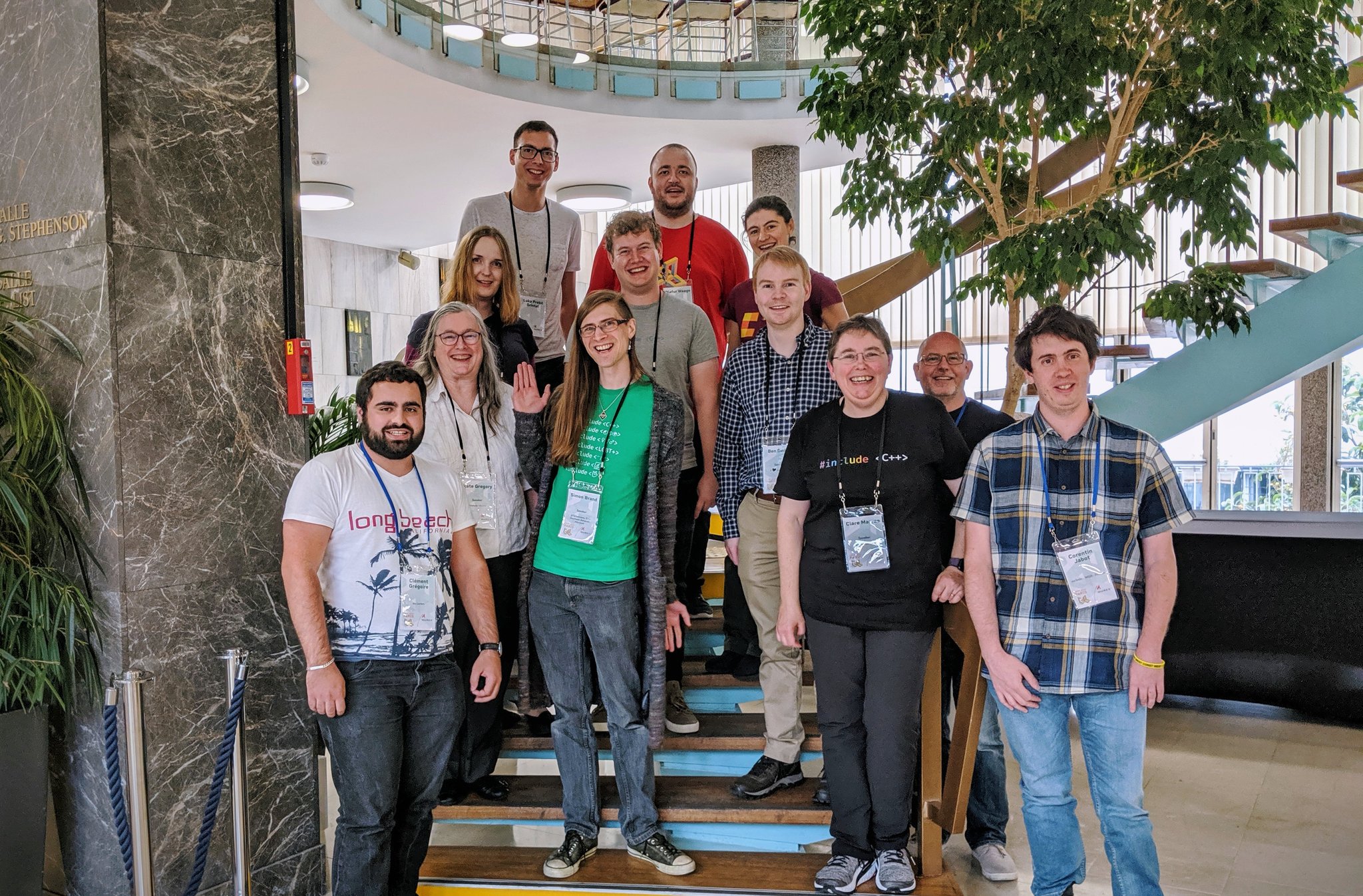
We’ve seen these shirts at conferences and user groups around the world, at C++ standards meetings, and on a lot of speakers and influencers. We think they send a strong message to attendees that the world is full of friendly and welcoming people who will not exclude you because you are different in some way. If you want one, we have a US-based store and a European store, or you can find us at a conference near you. We try to diffuse the stickers around the world – if you run a user group and are going to be at a conference, get in touch with one of us (the conference channel on our discord would be the best place) to see if you can get a handful of stickers to take home and give out at the group. Last year at about this time, someone asked if we were interested in partnering with the Women in Tech Fund to get women to CppCon. We sure were! The conference donated tickets at below their catering costs, and we raised $4000 to cover travel and accommodation for our scholarship winners. It was a big success and we keep doing it at conference after conference. Right now we’re raising for CppCon again – this year not just women, but anyone who is under-represented in the C++ community, can apply. If your employer isn’t sending you, why not see if we can? 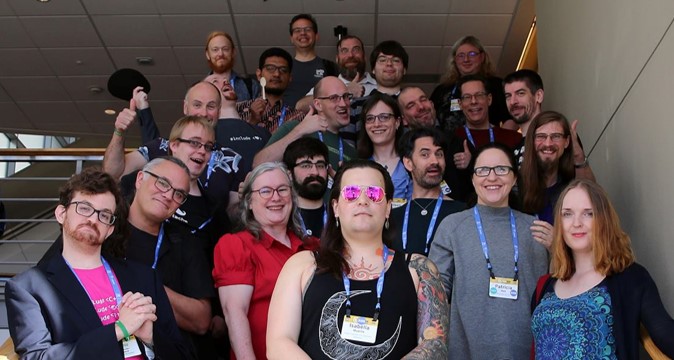
This is a lot more than we had originally planned to do. A number of us started insisting on a Code of Conduct before agreeing to submit talks to a conference, and suddenly it seems all the C++ conference have good Codes of Conduct now, with real enforcement too. There’s a best practice gaining popularity of introducing the Code of Conduct team at the start of the conference too. We started handing out pronoun stickers to put on badges, and not only do lots of people take them (please take one even if your gender is obvious, it makes life easier for those whose isn’t) but some conferences have even started including a pronouns field on badges. We’ve built this amazing friendly community on the discord where people are learning and growing and becoming leaders in the C++ community at large. We’ve seen talks and demos and forms and web sites changed after we pointed out that a particular wording or example wasn’t welcoming and inclusive. People generally want to be welcoming and inclusive, they’re just not sure how to do it, so our strategy of providing really specific unsolicited advice has worked well. And probably the thing I’m most proud of is the people – actual breathing humans – we have sent to conferences. Going to a conference is career-changing, especially when you’re relatively inexperienced. You can meet your heroes, ask questions, learn a ton, make connections, get advice, and re-energize your connection to this industry and your job. Already I am seeing former scholarship winners on stage, donating to the current fundraiser, and finding their voices on Twitter and our discord. It’s amazing. I want to pinch myself some days. The people who form the core of #include support and encourage each other. Many of us have given talks we would never have otherwise given. I won’t speak for her, but I expect the jaw dropping and enlightening Deconstructing Privilege talk that Patricia Aas has been giving could be one of them. If you haven’t watched it, you should. A lot of what we’re doing at #include is “privilege lending” – using our positions to ask for things to make people with less privilege feel welcome. We’re also teaching people who’ve been spared some hardships about the realities some other people face. Often this is all it takes for things to change quite quickly. We’ve also done a lot of lightning talks and internal corporate presentations about #include and what we’re trying to do, but it seems like none of them ever get recorded and uploaded. Rest assured, we’re still working hard to move the needle when it comes to inclusion in the C++ community. What’s next? Well, we’d love to start seeing child care available at C++ conferences. We’d love to see other developer communities doing some of what we’re doing, and we’re going to keep learning from other developer communities too. We’re seeing things like quiet rooms, pronouns on conference badges, and food labelling becoming the norm. And we’d love to get suggestions from anyone who feels excluded from conferences, training, job opportunities, and online communities. Join the discord and join the conversation, or find us on Twitter. See you there!
Kate
© Copyright 2025 Kate Gregory
Theme design by Bryan Bell
newtelligence dasBlog 2.3.9074.18820  | Page rendered at Friday, 25 April 2025 09:25:44 (Eastern Daylight Time, UTC-04:00)
|
On this page....
| Sun | Mon | Tue | Wed | Thu | Fri | Sat |
---|
26 | 27 | 28 | 29 | 30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 |
Pluralsight Free Trial
Search
Navigation
Categories
Blogroll
Sign In
|