 Monday, 30 June 2008
Justin Etheredge made an interesting point about code readability. He asks us to compare these two functions: public static int GetNextSize(int i)
{
//multiply it by four and make sure it is positive
return i > 0 ? i << 2 : ~(i << 2) + 1;
}
public static int GetNextSize(int i)
{
return Math.Abs(i * 4);
}
They do the same thing. One might be faster to execute if there was no such thing as an optimizer. But bit twiddling is notoriously hard to read and maintain. In the comments you can see people saying "don't those two actually return different values?" And that's really his point. Human beings have to read your code and maintain it. They have to understand it. And if you pre-optimize, if you decide that bit shifting is faster than multiplying, you make like harder for everyone after you. Since that might include you yourself, a few years from now, think twice about it. Write readable code. Let optimizers optimize. People time is way more expensive than CPU time.
Some folks think this is a good rule for staff, but that consultants who charge by the hour should go with the clever code. Generally they have two reasons for this. First, they worry that if they write the simple code, someone will look at it and ask "how much an hour did I pay for that!?!?!". I don't fuss about that, I just think "one dollar for hitting it with a hammer...". Second, they look forward to being brought back every time any changes need to be made to that code, since they're the only ones who understand it. I think that's shortsighted. Once the client realizes you've done that, you'll never get to work on any new projects for them. I'd rather work on a steady stream of new stuff than be stuck bugfixing old stuff for people who resent that I tricked them with my cleverness. And when I do have bugfixing to do, I appreciate being able to read what I wrote .
Kate
 Sunday, 29 June 2008
Lately some people I know have been revisiting the "why are so many Microsoft samples in C#" question. They are VB programmers, and they're just not feeling the love. Man, I know how that feels . Several recommendations of Instant VB reminded me that I had been meaning to try Instant C++. This is a $139 product that converts C# to C++/CLI (there is also a version to convert VB to C++.) There's a demo available, and it serves as an excellent example of what is both good and bad about code converters. Here's a comparison of the source and converted code for a demo I use to illustrate UAC in Vista programming:

Sure, it's boring as all get-out to change string to System::String^, though I would probably have done a using for the namespace and just said String^. (In fact, there's a using namespace System; in there already, but the converter doesn't seem to take advantage of it by omitting namespaces.) But there is so much here I don't like. First, I'm a String^ s person, not a String ^s person. Then there's how it handles the using. Hello? Stack semantics anyone? No? And where's my project file? I pointed this at a .csproj file, but I don't seem to get a .vcproj file in return, so I'll need to create a project and add the converted code into it. That's probably ok if I just want to convert sample code to paste into my real project, slightly less ok if I wanted to convert the sample project and test it.
Still, if you're using a relatively new technology, and you need to get to it from C++/CLI because you're writing a wrapper for legacy code or the like, and you get SOOOOO BOOOORED going through samples changing . to :: and new to gcnew and adding ^, then this is a cool tool to save you hours of that kind of thing. Just don't skip the step where you actually make it read like proper C++ code.
Kate
 Saturday, 28 June 2008
Because I graduated from the Faculty of Engineering at the University of Waterloo, I got an email about their participation in Go Eng Girl!, a province-wide initiative to show girls in Grades 7 through 10 what engineering is all about. If Waterloo isn't the closest university to you, go ahead and see if something nearer is also participating. I suspect, though, that many of us would like our daughters to take engineering at Waterloo if they're to take it anywhere - the reputation is excellent. (Remain calm if you support another institution; I also have a graduate engineering degree from Toronto and have worked alongside excellent engineers from a variety of universities, so let's not go there. And no, I haven't forgotten the weather in Waterloo.)
Looks like you can register online just before school starts again in the fall. Bookmark the site and check it out closer to the date.
Kate
 Friday, 27 June 2008
Seriously, once you have installed SP1 of Vista, if you have any more troubles with it, you can get technical support for free.
Free unlimited installation and compatibility support is available for Windows Vista, but only for Service Pack 1 (SP1). This support for SP1 is valid until March 18, 2009. Chat and e-mail support is available only in the United States and Canada.
(Phone support I guess is available worldwide.)
If something still won't work for you on Vista, drop them an email or open a chat session, and get it sorted out. It's free, so why not?
Kate
 Thursday, 26 June 2008
This delightful article by Maria Blees slipped by me during my most recent blogging hiatus. It presents a handy framework to run unit tests on native code, some macros to hook you into VSTS code coverage, and some excellent guidance on the reality of testing and refactoring. It also recommends some nice resources. (How fun is it to read a plea (in a pdf she recommends) for designing to be testable and not to put real code in your event handlers, then realize it's from six years ago?) If you write C++ code, go read this article now. It has plenty of real meat in it, and concludes with this paragraph:
My goal has been to show that unit testing native C++ can be easy, fun, and you can get started right now. To give you an idea of the power of WinUnit, take a look through the TestWinUnit project, where I used WinUnit to test itself. Those examples are completely real world and will show you advanced usage you can apply to your own unit tests. If you've been struggling with your native C++ unit testing, WinUnit makes it easy—and any time you can make testing easy, you're far more likely to actually do it.
Mission accomplished, Maria!
Kate
 Wednesday, 25 June 2008
Before Vista, you could right-click a shortcut, executable, or whatnot and choose Run As ... to run that item as another user. 99 44/100 of the time you wanted to run it as your administrative account, if you were one of the 3 people on the planet who had an administrative account. Occasionally, you were borrowing someone's computer and wanted to run something that relied on Windows integrated security, for which you wanted to be yourself. And more relevantly for me, you might want to demonstrate how Windows integrated security worked, say by having 3 or 4 browsers open that were all authenticated (to Windows itself) as different users.
So if any of that rings a bell for you, you want ShellRunAs. It's free, it's from Microsoft, and it works on Vista.
Kate
 Tuesday, 24 June 2008
P/Invoke (aka DllImport) signatures are not the world's most fun things to create. You start with a native declaration, and then hand-map native types to the equivalent (you hope) managed types. If at all possible, you head to www.pinvoke.net and look up the API you are calling and paste in whatever some kind soul put on the wiki. If not, well you have some mechanical work to do.
But now the Interop team has a little tool for you! Look up the API you want, choose a language, click the Generate button and -tada!- your declaration is ready to be copied and pasted. (Even has doc comments explaining the params.) Not calling a well-known API (maybe it's your own code from a native project?) No problem, paste in your native C++ signature and translate. Need to go the other way around (what native signature corresponds to a managed one?) No problem.
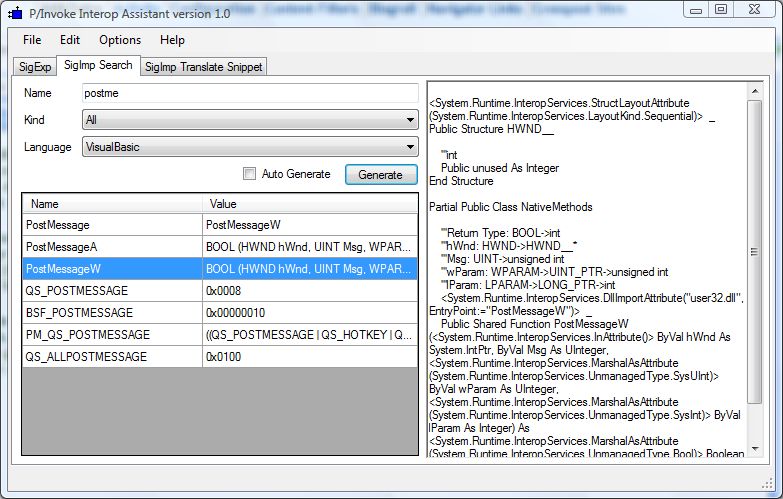
Sweet. The code it generates isn't pretty (for example it doesn't add any using/Imports statements, so everything gets the full dotted name every time, and everything is explicitly declared) but then again, who reads generated code? Stick a comment in front of it saying you generated it and leave it alone. Even if it needs a little hand tweaking now and again (and I honestly don't know whether it does or not) it will still save a TON of time. It's on CodePlex, so go get it.
Kate
ps: The list of APIs is in a XML file, and I noticed the Vista-only ones I tried (restart and recovery related mostly) weren't found, so if you wanted to make a contribution to the project...
 Monday, 23 June 2008
I said I would post it when I got it.
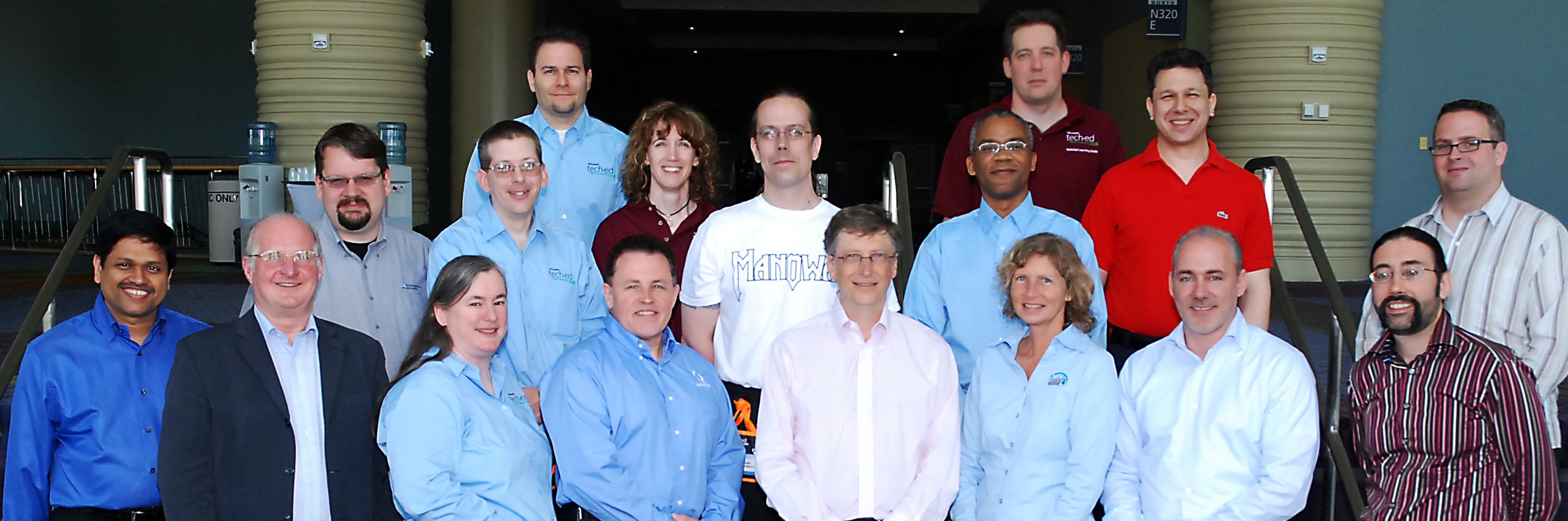
Wow. Almost as amazing to me as being in a picture with Bill is being friends with so many of the others in the picture. What a day that was.
Kate
ps: I know it looks like most of us are all wearing the same light blue shirt. We're not. Stephen, Scott, and I are all wearing Tech Ed speaker shirts, because we were speaking that day. Across the front row, Dave is wearing his Culminis shirt (it's a slightly different blue) and Morgan her INETA shirt. Dan is actually in a white shirt that is reflecting Morgan's shirt. Unfortunately I can't remember whether John (behind Morgan) was wearing a speaker shirt for sure, but I think so.
Double ps: updates from Rob Zelt and John Holliday.
 Sunday, 22 June 2008
So imagine you have some managed code, and it calls some other managed code, and that lower level code tries to do something that requires a certain level of code access security. Maybe it wants to write to the files system, or open a network connection, for example. You probably know that there is a stack walk to make sure that everyone has the appropriate permissions. But what if that stack includes some native entries? Managed code called native code (maybe with P/Invoke; maybe with IJW-style C++ interop) and then the native code called managed code (probably with COM interop though there is also "reverse P/Invoke" I suppose. What happens then? Shawn Farkas knows. The whole blog is good reading if Code Access Security and the way the runtime actually works are things you should know more about, but haven't investigated. Bite size pieces of intruiging questions, along with definitive answers. Nice.
Kate
 Saturday, 21 June 2008
When I interview people, I throw them lots of softball questions. One of them is "why should I hire you?". Some people think this is a scary and difficult question. On the contrary, it means "here is your invitation to tell me why you are right for this job. Feel free to brag as much as you like. I will now listen while you tell me what is good about you. Extra credit if you have some clue about what is valuable to me." Interview questions just don't get any softer. Yet some people blow it. They answer a different question, usually "why is it important to you that you can work here?" but occasionally "why do you want to be employed?". Fascinating information may come out of your mouth, but it isn't what I asked for. This of course means that I learn something else from your answer - something about your attention to detail, your ability to think of my needs instead of your own (and if you join us, to think of client needs), and your grasp of the business picture here.
Another question that terrifies people is "tell me your biggest weakness." I generally don't ask this because you just get "well I am a perfectionist, I must have all my work complete and beautiful" or "I work too hard I am so devoted" or "my brilliance shines so brightly that it scares others" or the like - bragging disguised as modesty. I already have a question which prompts you to brag. But the other thing is that not all weaknesses are things you actually need to improve or fix. For example, I sing poorly. I have arranged my life so that this doesn't matter. I would never raise it in a job interview. (If I was interviewing for Canadian Idol, it would be a big deal though, wouldn't it?)
If you do get this question, you want to provide an answer that is honest, that identifies something relevant to your workplace, and where you can say what you are doing about it, but more importantly what you need from them about it. "I work long hours and can tire myself when I get caught up in an exciting project. I really value firms that track overtime hours and give me an extra day off from time to time to acknowledge those long days and let me recharge my batteries." "I have very high standards and when I was younger every client got a Cadillac even if we bid a Pinto. I have learned the importance of requirements and project plans to keep my work in scope and make sure I solve the problem we are tackling in the time we have allowed, but it's still a weakness that I can be vulnerable to scope creep." "I think very quickly and solve problems faster than most people, but I can forget to make sure my colleagues are with me and that we have consensus. I work best in an atmosphere where at least one person can keep up when my mind takes off, and remind me to explain my thoughts to everyone." These answers genuinely reveal something about yourself that would be a fatal flaw in some companies. The thing is, if they're true (and not something you made up thinking this was the "please brag" question) then you don't want to work in those companies. Revealing this weakness will save you from a miserable job. They also set a requirement on the interviewer. Will you give me time off when I have been working long hours? Will you have the discipline and project management to help me stay in scope? Am I doomed to be the smartest person in the room every time? Let the firm assure you (if they can) that they will be a good fit for you.
Take some time to think about your true weaknesses. Lack of experience, impulsive or overly rigid actions, ignoring procedures or refusing to ever deviate from them, freezing out slower thinking colleagues or never making a decision or offering an opinion without checking with everyone else first, skillset that's a little old fashioned, slow learner, new thing junkie, continuous partial attention, tendency to go dark, hesitant to ask for help, afraid of public speaking, horrible grammmar and spelling, everyone has something. Take your time and construct a reply that honestly states your true weakness, then explains the environment you need to thrive given that you are this kind of person. If you like, include something about how you are strengthening that part of yourself already, if you are. Or if that's just who you are (like my singing) don't pretend you plan to fix it. Resolve not to work for firms that can't support you in your weak areas. Never pretend just to get employed.
Kate
© Copyright 2025 Kate Gregory
Theme design by Bryan Bell
newtelligence dasBlog 2.3.9074.18820  | Page rendered at Thursday, 03 July 2025 22:22:36 (Eastern Daylight Time, UTC-04:00)
|
On this page....
Pluralsight Free Trial
Search
Navigation
Categories
Blogroll
Sign In
|